What Is Storybook in React Native?
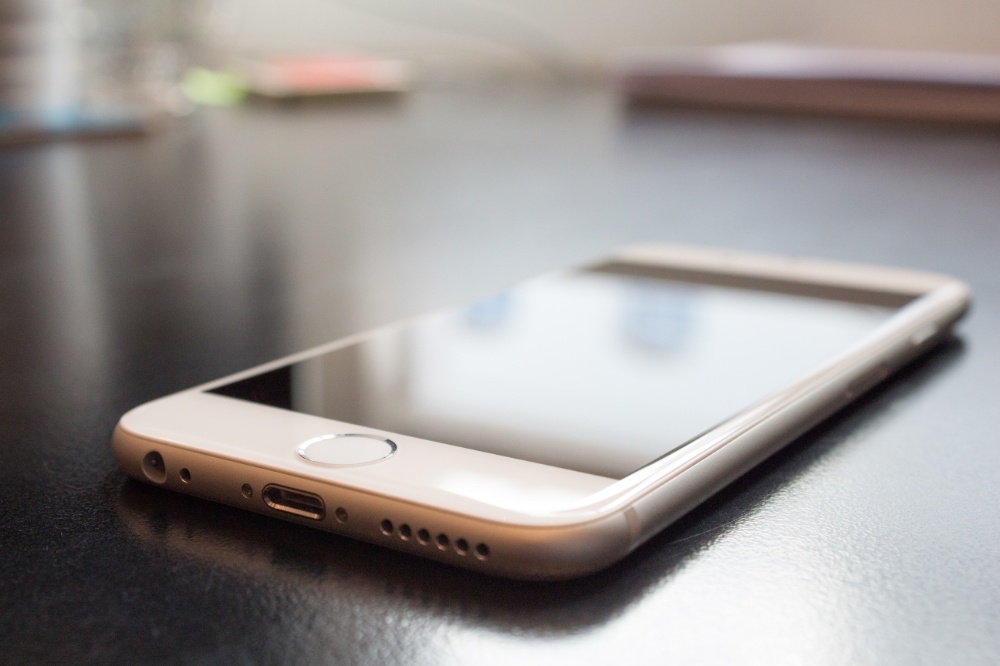
The robust tool runs outside the main app and allows developers to create components independently.
At Netguru, we use a design system based on React Native, and one of the tools we use for design system implementation in React Native development is Storybook. An important aspect of branding is coherent design: Storybook in React Native offers a foundation to achieve that, saving you time, reducing errors, and boosting satisfaction.
Continue reading for the lowdown on Storybook in React Native, including advantages and setup.
Storybook - a definition
In the words of Storybook themselves: “Storybook is a tool for UI development. It makes development faster and easier by isolating components. This allows you to work on one component at a time. You can develop entire UIs without needing to start up a complex dev stack, force certain data into your database, or navigate around your application.”
The open source tool streamlines UI development, testing, and documentation, and is made for React Native, React, Vue, Angular, Web Components, Ember, HTML, Mithril, Marko, Svelte, Riot, Preact, and Rax. By offering a sandbox to build interfaces in isolation without fussing with data, APIs or business logic, it’s possible to develop edge cases and hard-to-reach states.
Advantages of Storybook
Storybook is a powerful tool that’s quick to install. Other substantial benefits include:
- Easy access to all components – access each component and browse through its states without having to worry about business logic
- Increased chance of catching all edge cases
- Ease of sharing and reusing components
- Improved code quality – because you write components in isolation, disregarding business logic, you potentially put greater emphasis on code quality and reusability
- Better documentation
Do we suggest using Storybook in every new project?
Yes!
Having a project with a big library of components and not using Storybook may lead to situations where developers (especially new or returning to the project) spend more time than necessary on searching for specific components.
Creating a new story isn’t time-intensive or resource-heavy. Using custom emmet shortcuts, stories are quick, easy, and bring great benefits. All you need to do is add the story to a default development flow, and build and test components.
Setup
We’ve outlined what Storybook and stories are, and the advantages, so now it’s time to get a bit more technical. Let’s move on to setup and a demo.
1. Installation
npx -p @storybook/cli sb init --type react_native
2. Storybook runs in the place of our application, so we have to change AppRegistry
// index.js
let RegisteredApp = App;
RegisteredApp = __DEV__ ? require('./storybook').default : App;
// ^ comment this line if you don't want to use Storybook
AppRegistry.registerComponent(appName, () => RegisteredApp);
3. Run the application (in 3 terminal tabs)
yarn storybook
yarn start
yarn <platform>
You should end up like this:
Detecting your stories from any directory
Storybook only shows you stories that are included inside the configure function inside storybook/index.js. Unfortunately, you can’t configure Storybook so it searches for stories on its own, but here’s something to help react-native-storybook-loader
After you call yarn prestorybook
in your terminal, that command will look for any
.stories.js files in the components/** directory (in this case), and it will add them to the configuration.
Setup
1. Installation
yarn add -D react-native-storybook-loader
2. Configuration inside package.json
{
"scripts": {
"prestorybook": "rnstl"
},
/* ... */
"config": {
"react-native-storybook-loader": {
"searchDir": ["./src/components"],
"pattern": "**/*.stories.js",
"outputFile": "./storybook/storyLoader.js"
}
},
}
3. Change storybook/index.js
// ...
import { loadStories } from './storyLoader';
configure(() => {
loadStories();
}, module);
4. Run script
yarn prestorybook
Now, you can create a story inside your component directory, run yarn prestorybook
, and it will be added to configuration automatically, like in example below:
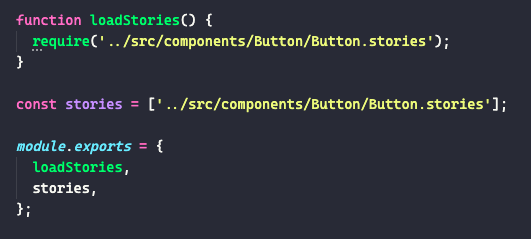
storybook/storyLoader.js
storybook/stories dir is now useless, so you can delete it.
Creating a story of a component
├── components
│ ├── Button
│ │ ├── Button.js
│ │ ├── Button.stories.js
Button.js
import React from 'react';
import { StyleSheet, Text, View, TouchableOpacity } from 'react-native';
const Button = ({
label,
onPress,
style,
textColor = '#ffffff',
backgroundColor = '#95C4CB',
fill = false,
...rest
}) => (
<TouchableOpacity
{...rest}
onPress={onPress}
activeOpacity={0.75}
style={fill ? { flex: 1 } : {}}
>
<View style={[s.wrapper, { backgroundColor }, style]}>
<Text style={[s.label, { color: textColor }]}>{label}</Text>
</View>
</TouchableOpacity>
);
export default Button;
const s = StyleSheet.create({
wrapper: {
height: 45,
alignItems: 'center',
justifyContent: 'center',
borderRadius: 30,
},
label: {
fontSize: 14,
fontWeight: '500',
},
});
Button.stories.js
import * as React from 'react';
import { View, StyleSheet } from 'react-native';
import { storiesOf } from '@storybook/react-native';
import { action } from '@storybook/addon-actions';
import { object, text, color, boolean } from '@storybook/addon-knobs';
import Button from './Button';
storiesOf('Button', module)
.addDecorator(story => <View style={s.decorator}>{story()}</View>)
// 👇 you can add multiple variants of component, here's variant with name 'default'
.add('default', () => (
<Button
onPress={action('onPress')} // 👈 action
// 👇 knobs
label={text('label', 'Button label')}
style={object('style')}
textColor={color('textColor', '#ffffff')}
backgroundColor={color('backgroundColor', '#95C4CB')}
fill={boolean('fill', false)}
/>
));
const s = StyleSheet.create({
decorator: {
flex: 1,
justifyContent: 'center',
padding: 16,
},
});
Actions
Use actions to display data received by event handlers in Storybook. How does the addon work? By supplying Storybook-generated ‘action’ arguments (args) to stories. The two ways to generate an action argument are action argType annotation and automatically matching args.
Knobs
The knobs addon is a first-rate resource that allows developers and designers to edit props dynamically using the Storybook UI. It allows you to play with components in a controlled environment, calling them one by one according to the fields you want to control.
Knob types available in Storybook
- text
- number
- boolean
- color
- date
- array
- object
- files
- button
- radios
- select
Summary
UI development tool Storybook makes testing, documentation, and knowledge-sharing easy, offering an easy-to-use solution that improves code quality and enables better documentation. Other benefits include easy access to all components without having to worry about business logic, increased chance of catching edge cases, and ease of sharing and reusing components.
Moreover, with quality addons such as actions and knobs, you can expand Storybook’s UI and behavior, adding to the user experience. As such, we suggest using Storybook and stories in every new project.