Accessibility in React Native
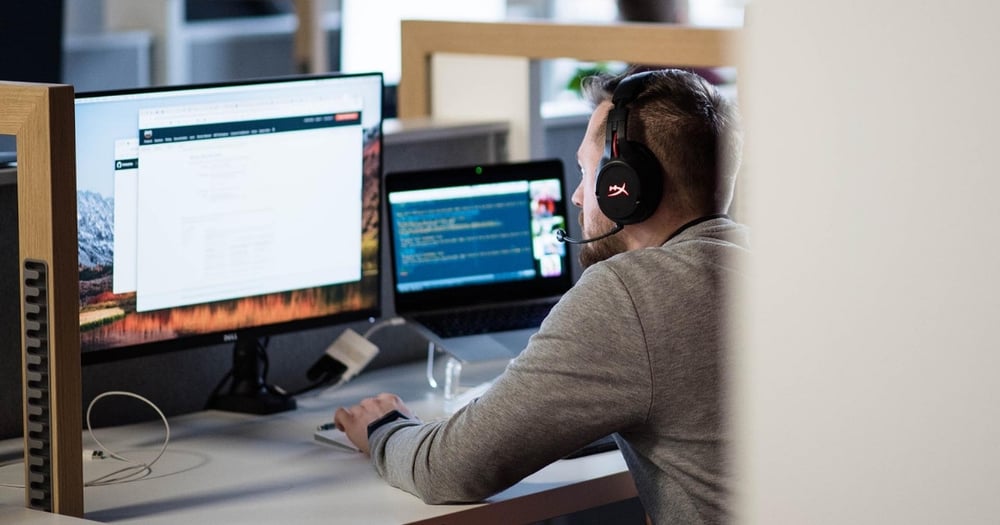
You can activate them anytime in your device’s settings. Both applications work similarly with minor differences, but the main concept is to read everything that is on screen aloud.
It's quite helpful for blind people to know what's going on and how they can interact with your application. The good news is React Native supports accessibility fairly well. There is even a nice guide in this doc.This is why I won't make this article long - it will be mostly based on this guide. Another good thing about this is the ease of implementation. You can create a well-supported application in terms of accessibility without any trade-offs and with minimal effort.
How these accessibility applications work
1 tap on an accessible element - the app will mark the component area with a border and then read the content inside it aloud.
2 fast taps on an accessible element - if the element works as a button (has an onPress property), it will trigger that method.
Note: By default, all touchable elements (text, touchables, and buttons) are accessible.
Make inaccessible elements accessible
Just add the accessible={true} property to the desired component.
<View accessible={true}></View>
Examples
In the example below, we create a button by using the TouchableOpacity component that is already accessible. It is also a clickable element for the user. This means that TalkBack/VoiceOver will point this out to the user while reading this component.
An additional property, accessibilityLabel, is used to let the user know the role of this element. The output of the application will be something like this: "This a button to open a modal. Double click to activate". The text component inside TouchableOpacity won't be read if accessibilityLabel is used. Otherwise, if no accessibilityLabel is attached, text inside the Text component will be read.
<TouchableOpacity
accessibilityLabel="This is button to open modal"
style={styles.touchable}
onPress={this.openModal}>
<Text>React Native</Text>
</TouchableOpacity>
If we need to add an onPress property to an element that is not clickable by default, we can add the accessibilityRole property to mark this element as clickable (some action takes place after it is clicked). TalkBack/VoiceOver will mention that while reading this component. Firstly, it will read the text inside and then mention that this it is clickable. The final output would be something like this: "React Native. Button"
<Text accessibilityRole="button" onPress={this.openModal}>React Native</Text>
In this case, we wrap 2 Text elements inside a View that is accessible. Also, there is an accessibilityLabel property. This means that TalkBack/VoiceOver will treat View as one component without access to the selected Text elements.
This is caused by an accessible property value set to true. By adding the accessibilityLabel property we suggest to the application to read this label instead of the 2 Text elements inside.
<View accessible={true} accessibilityLabel="There are fruits">
<Text>Apple</Text>
<Text>Orange</Text>
</View>
Detecting if the device has a screen reader currently active
We can use the AccessibilityInfo API to check if a screen reader is available on a user device. There is a simple usage example below.
componentDidMount() {
AccessibilityInfo.addEventListener(
'screenReaderChanged',
this.handleScreenReaderToggled,
);
AccessibilityInfo.isScreenReaderEnabled().then(screenReaderEnabled => {
this.setState({screenReaderEnabled});
});
}
componentWillUnmount() {
AccessibilityInfo.removeEventListener(
'screenReaderChanged',
this.handleScreenReaderToggled,
);
}
handleScreenReaderToggled = screenReaderEnabled => {
this.setState({screenReaderEnabled});
};
How to enable VoiceOver (iOS) and TalkBack (Android)
Both can be found in general system settings. For the exact place check out the documentation.
Other features
There is also a lot more we can get from Accessibility in React Native. I didn't mention everything because there are various apps we create and each has different needs. For specific methods and properties, I direct you to the well-written accessibility documentation.
My point of view and final comment
From my view, with a low dose of effort, you can create a quite well-written accessible application. Just keep attaching key features (properties) to components, which from my perspective are:
- accessible - e.g. if you think that a View element is important and should be described with its associated accessibilityLabel element
- accessibilityLabel - to inform the TalkBack/VoiceOver application how to describe a specific element
- accessibilityRole - to inform TalkBack/Voiceover about the purpose of this element (with an emphasis on the "button" value if you make non-standard touchable elements clickable)
- accessibilityValue - if you use sliders or progress bars
- accessibilityHint - if the label is not enough and you want to provide further explanation
Although there are many other properties and methods that you can use depending on your needs, keep this in mind if you want to make your app really well-written in terms of accessibility.