API Endpoints Protection Using JWT
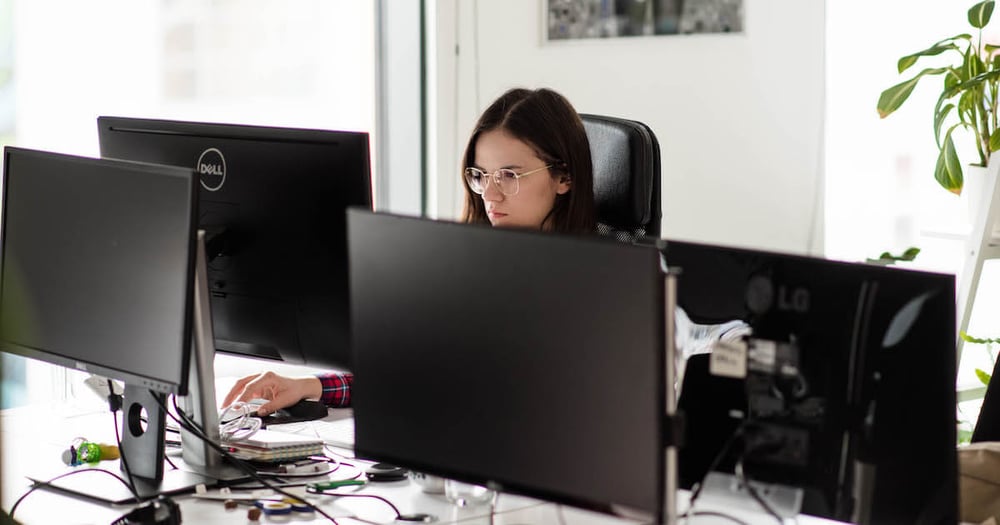
APIs act as an intermediary between the two applications that allows them to communicate with each other.
Nowadays, APIs have become more and more popular and widely used in many applications. However, with the increase in the use of APIs, the security risks are also increasing. API endpoints are often the target of bots or hackers who are looking to exploit vulnerabilities.
One of the most common ways to protect API endpoints is by using JSON Web Token (JWT). JWT is a type of token that is used to authenticate users. It consists of three parts: header, payload, and signature.
The header contains information about the type of the token and the algorithm that is used to generate the signature. The payload contains the actual data that is to be passed to the API endpoint. The signature is used to verify the authenticity of the token.
JWT is a very popular way to protect API endpoints because it is easy to implement and it is very effective in preventing attacks.
What is JWT?
JWT is short for JSON Web Token. It is used to transfer data in the form of a JSON object that is securely signed using a JSON web signature (JWS) and is optionally encrypted using JSON Web Encryption (JWE).
Why use JWT?
JWT provides a way to securely transfer data between two parties, typically between a server and a client. By signing the data, the receiver can be sure that the data has not been tampered with. And by encrypting the data, the contents can be kept confidential.
How does a JWT work?
A JWT typically contains a header, a payload, and a signature. The header and payload are JSON objects, which are Base64Url encoded and joined together with a period “.” . The signature is generated using the header and payload, along with a secret key.
Here is an example of a JWT:
eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJzdWIiOiIxMjM0NTY3ODkwIiwibmFtZSI6IkpvaG4gRG9lIiwiYWRtaW4iOnRydWV9.TJVA95OrM7E2cBab30RMHrHDcEfxjoYZgeFONFh7HgQ
When using a JWT to protect an API endpoint, the client first makes a request to the server to authenticate. The server then responds with a JSON object that contains a JWT. The client then includes the JWT in subsequent requests to the server.
To verify the JWT, the server:
- Reads the JWT from the request header
- Base64Url decodes the header and payload
- Verifies the signature using the header and payload, along with the secret key
- If the signature is valid, the server processes the request; otherwise, the server returns an error
When to use a JWT?
JWT is a useful tool for protecting API endpoints. When using a JWT, the client first authenticates with the server. The server then responds with a JWT. The client then includes the JWT in subsequent requests to the server. The server can then use the JWT to verify the identity of the client.
JWTs can also be used to protect data that is being stored on the client. For example, a JWT can be used to encrypt the contents of a cookie.
What are the benefits of using JWT?
There are several benefits to using JWT:
- JWT is a standard format that is supported by many different libraries
- JWT can be used to protect API endpoints
- JWT can be used to store data on the client
- JWT is a relatively simple technology that can be easily implemented
What are the drawbacks of using JWT?
There are some potential drawbacks of using JWT:
- If the secret key is compromised, the attacker can generate their own JWT and access the API endpoint
- If the data is not encrypted, it can be read by anyone who has access to the JWT
- JWT should not be used to store sensitive data, such as user passwords
How to use JWTs to protect your API?
JWTs can be used to protect your API in two ways:
- Authentication: You can use JWTs to authenticate users before allowing them to access your API. This is done by validating the JWT that the user sends in the Authorization header of their request.
- Authorization: You can use JWTs to authorize users to access certain API endpoints. This is done by validating the JWT and checking if it contains the necessary permissions to access the endpoint.
Protecting your API with JWTs is a simple and effective way to keep your data safe.
Why is JWT a good choice for API endpoint protection?
If you're looking for a way to protect your API endpoints, JWT is a great choice. JWT is an open standard that defines a compact and self-contained way for securely transmitting information between parties as a JSON object. This information can be verified and trusted because it is digitally signed. JWT is used in many different applications, including API endpoints.
JWT provides a number of features that make it a good choice for API endpoint protection:
- It is lightweight and fast
- It is standards-based
- It can be used in a stateless manner
- It is easy to implement
In addition, JWT has some great libraries available that make it even easier to use. So if you're looking for a way to protect your API endpoints, JWT is definitely a good option.
How to Implement JWT?
JWT can be implemented using different libraries, such as the JWT〈https://jwt.io/〉 library.
To use JWT, the following steps need to be taken:
- Generate a secret key
- Create a JWT using the secret key
- Send the JWT to the client
- The client includes the JWT in subsequent requests
- The server reads the JWT from the request header
- The server Base64Url decodes the header and payload
- The server verifies the signature using the header and payload, along with the secret key
- If the signature is valid, the server processes the request. Otherwise, the server returns an error.
JWTs are typically used in web applications and APIs to authenticate users and validate their permissions.
When a user logs in, they are given a JWT which they can then use to make authenticated requests to the API. The JWT will contain information about the user, such as their name and ID.
To authenticate a request, the user simply sends their JWT in the Authorization header of the request. The API will then validate the JWT and, if it is valid, allow the request to continue.
If the JWT is invalid, the API will return an error and the request will be rejected.
There are a few different ways you can implement JWT-based authentication for your APIs. One popular way is to use the Auth0 service. Auth0 provides an easy-to-use platform that allows you to quickly add authentication and authorization to your APIs.
Another way to implement JWT-based authentication is to use the jwks-rsa library. This library allows you to create and verify JWT tokens using the RS256 algorithm.
Whichever method you choose, make sure that you understand how JWT works and that you keep your secret key safe and secure.
How to implement JWT in a Node.js API?
A JSON Web Token(JWT) is an open standard that defines a way for transmitting information between two parties in a JSON object. This information can be verified and trusted because it is digitally signed. JWTs can be signed using a secret or public/private key pair.
In order to use JWT in a Node.js API, we need to install the jsonwebtoken module.
npm install jsonwebtoken
We can then require the module in our app.js file.
var jwt = require('jsonwebtoken');
We can create a token by passing the payload and the secret to the sign method.
var token = jwt.sign({ foo: 'bar' }, 'secret');
We can then verify the token by passing it to the verify method.
jwt.verify(token, 'secret', function(err, decoded) { console.log(decoded) }); // bar
That's all there is to using JWT! By using JWT, you can be sure that your API endpoints are secure and can only be accessed by authorized users.