How to Make Your App Respond Gracefully to Region Change
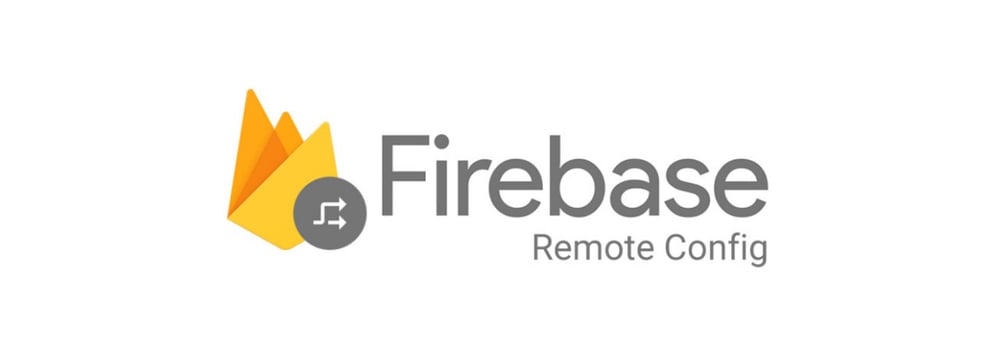
For example, you may want to display different content in some regions or change the color palette depending on the user’s location. Today I will show you how to do it using Firebase Remote Config and our great Sticky Parallax Header library!
First, let’s talk about Firebase Remote Config. It’s a really cool functionality of the Firebase “stack” which you can implement in your app in no more than a few hours. It can boost your development speed if you use it early - later in the development process you can use it to automatically configure the app from the server, without needing users to update it via Google Play Store or App Store! Moreover, you can personalize your app depending on region or some other variable. All of this is completely free, so let’s move on to the implementation in React Native!
As I said earlier, I will show you the possibilities offered by Firebase Remote Config using the Sticky Parallax Header library and the example app that comes with it, which you can find here: https://github.com/netguru/sticky-parallax-header. We can easily extend its functionality with Remote Config, which will allow you to change the app’s color scheme depending on location. In our case, let’s display one set of colors for users from Poland and other colors for the rest of the world. The first thing you will want to do is to install the react-native-firebase package along with the react-native-firebase/remote-config package.
The next thing you have to do is to create a RemoteConfigService that will initialize the react-native-firebase/remote-config library. Here’s how:
import remoteConfig from '@react-native-firebase/remote-config';
const RemoteConfigService = {
initialize: async () => {
await remoteConfig().setConfigSettings({
minimumFetchIntervalMillis: 300,
});
await remoteConfig().setDefaults({region: 'World'});
await remoteConfig().fetchAndActivate();
},
};
export default RemoteConfigService;
As you can see, I created an initialize method that will set the default remote config fetch interval to 300ms. This will enable me to test if everything works all right. In the production version you will probably want to fetch remote configuration less often. The next thing is setting default configuration values. Let’s assign the “World” value for our default region - it should change if we’re in Poland after the next method call. FetchAndActivate is responsible for fetching remote configuration and activating it inside our app. You have to call this method as soon as possible, so I recommend calling it in the App.js/App.tsx file.
The last step is getting a remote config inside the screen in which we will be changing the styling of our Sticky Parallax Header. But first things first - we have to create another method for it in our RemoteConfigService.
import remoteConfig from '@react-native-firebase/remote-config';
const RemoteConfigService = {
…,
getRemoteValue: (key) => remoteConfig().getValue(key),
};
export default RemoteConfigService;
Now let’s move on to HomeScreen, where I have our Sticky Parallax Header. We can place our code responsible for getting remote config values inside the componentDidMount method. After we get it, we have to set our state, so that the component will re-render. It can also be done in App.js and passed to the screen via navigation props, but let’s do it the other way:
componentDidMount() {
…,
const regionConfig = RemoteConfigService.getRemoteValue('region')?.asString()
this.setState({region: regionConfig})
}
Now we just have to create styles for our regions and the method that will apply colors to our Sticky Parallax Header. And that’s it! You just created an app that responds to region change.
getBackgroundColor = () => {
const {region} = this.state;
let style;
if (region === 'Poland') {
style = styles.homeScreenHeaderPoland;
} else {
style = styles.homeScreenHeader;
}
return style;
};
renderHeader = () => {
const {region} = this.state;
return (
<View style={[styles.headerWrapper, this.getBackgroundColor()]}>
<Image
resizeMode="contain"
source={require('../../assets/images/logo.png')}
style={styles.logo}
/>
</View>
);
};
Warning! Initialization of remote configuration is currently broken in react-native-firebase/remote-config version 8.1.1. You can downgrade the package to version 5 or wait for an update.