Node.js Memory Leaks: How to Debug And Avoid Them?
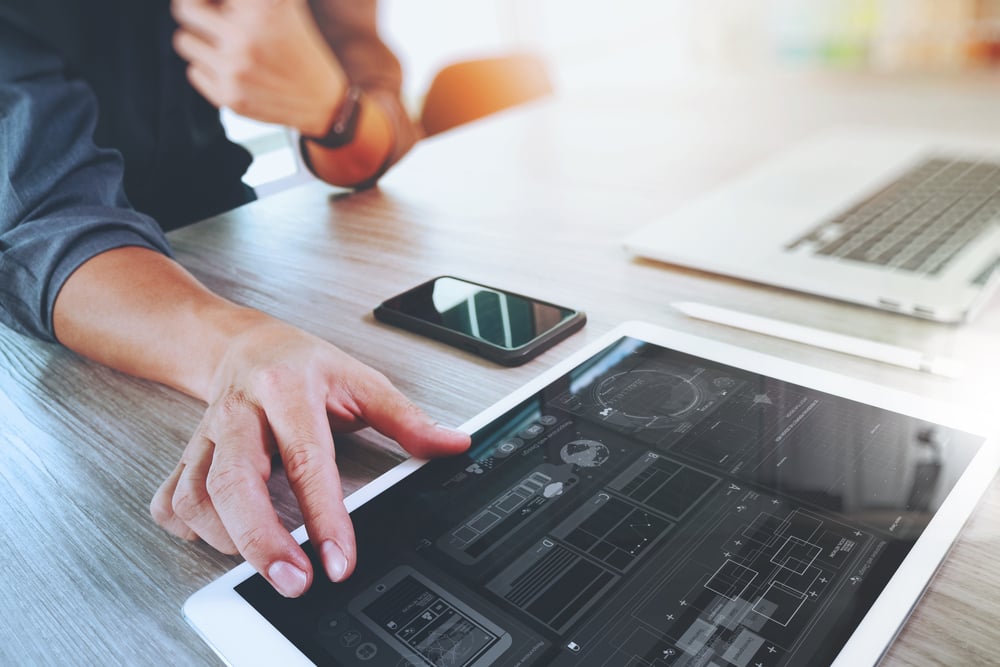
Understanding and mitigating memory leaks is crucial for ensuring the seamless operation of Node.js applications over time.
Memory leaks occur when a program fails to release allocated memory properly, leading to a gradual degradation of system resources and overall performance. In Node.js, which relies heavily on asynchronous, non-blocking I/O operations, identifying and resolving memory leaks becomes an essential skill for developers.
In this guide, we will delve into the intricacies of Node.js memory leaks, exploring not only how they manifest but, more importantly, how to effectively debug and prevent them.
Let's dive into the world of Node.js memory management, where understanding the nuances can make all the difference in crafting high-performance applications.
Memory Management in Node.js
In Node.js, memory management is crucial for ensuring optimal performance and efficiency. The V8 JavaScript engine, developed by Google and used by Node.js, employs automatic memory management through a process known as garbage collection.
Here are the key concepts related to memory management in Node.js:
1. Heap
The heap is a region of memory used for the dynamic allocation of objects during the runtime of a program. In Node.js, the heap is where objects, closures, and other dynamically allocated data reside. The V8 engine divides the heap into two main segments: the young generation and the old generation. Objects initially start in the young generation and are promoted to the old generation if they survive multiple garbage collection cycles.
2. Stack
The stack, in contrast to the heap, is a region of memory used for the execution of function calls and management of local variables. Each function call in Node.js creates a new frame on the stack, containing information about the function's local variables, parameters, and return address. The stack operates in a last-in, first-out (LIFO) manner, with each function call's frame being pushed onto the stack and popped off when the function completes.
3. Memory Allocation
In Node.js, memory allocation occurs when variables and objects are dynamically created during the execution of a program. When you create an object, the V8 engine allocates memory for that entity on the heap. The engine employs various memory allocation algorithms, including:
- Bump-Pointer Allocation: Allocates memory by moving a pointer up the heap, and when it reaches the end, it triggers garbage collection.
- Free List Allocation: Allocates memory from a list of free memory chunks, reusing previously allocated space.
4. Garbage Collection
Garbage collection is the process of identifying and reclaiming memory occupied by objects that are no longer in use. In Node.js, the V8 engine employs a generational garbage collector.
This collector divides objects into the young generation (where short-lived objects are allocated) and the old generation (where longer-lived objects are promoted). Garbage collection cycles, such as Scavenge for the young generation and Mark-Sweep-Compact for the old generation, help reclaim memory by identifying and collecting unused objects.
Understanding these fundamental concepts provides a foundation for comprehending how Node.js manages memory during the execution of applications. As you delve deeper into memory-related topics, this knowledge will prove valuable in identifying and addressing issues such as memory leaks and optimizing your Node.js applications for better performance.
Understanding Memory Leaks in Node.js
Memory leaks in Node.js can be sneaky issues that gradually make your application use more memory. This leads to performance problems and can eventually cause the app to crash. To tackle this, you have to understand what memory leaks are and how they occur in a Node.js environment.
Definition
In the context of Node.js, a memory leak occurs when the application retains no longer needed memory, preventing the Node.js garbage collector from reclaiming it. This leads to a gradual increase in memory usage, potentially resulting in performance degradation or, in extreme cases, application crashes.
How do Memory Leaks happen?
Memory leaks can occur in various ways in a Node.js application. Common scenarios include:
Unclosed Event Listeners
Event listeners that are not properly closed or removed can accumulate and cause memory leaks. Ensure that event listeners are detached when they are no longer needed.
Circular References
Circular references, where objects reference each other in a loop, can prevent the garbage collector from reclaiming memory. Detecting and breaking such loops is crucial to preventing leaks.
Unclosed Connections
Leaving database connections, file streams, or network sockets open without proper closure can lead to resource leaks. Always close connections after use.
Timers and Intervals
Repeatedly setting timers or intervals without clearing them can lead to memory leaks. Ensure that you clear intervals and timeouts when they are no longer needed.
Holding onto Unused Objects
Keeping references to objects in memory, even after they are no longer needed, can contribute to leaks. Be mindful of long-lived references and release them appropriately.
External APIs and Resources
Failing to release resources obtained from external APIs, such as third-party libraries or services, can lead to memory leaks. Follow best practices provided by these APIs for proper resource cleanup.
Remember that effective memory management is often about understanding the specific context of your application. Regularly monitoring and profiling your application's memory usage can help you identify and address potential issues. The goal is to cultivate good coding practices, promptly release resources when they are no longer needed, and use appropriate tools for debugging and profiling.
Debugging Memory Leaks
Identifying memory leaks in your Node.js applications is crucial for maintaining optimal performance. Fortunately, several tools and techniques are available to help you detect and diagnose memory leaks effectively.
1. Heap Snapshots
Heap snapshots are a valuable tool for identifying memory leaks in JavaScript applications, and they can be taken either manually from the Chrome Developer Tools, or programmatically using tools like the V8 heap snapshot feature. By capturing snapshots at various points during your application's lifecycle, you can compare memory usage and pinpoint objects that are not being released. Utilize the heap snapshots taken at different stages to analyze memory usage with tools like Chrome Developer Tools, which can highlight persistent objects across snapshots and indicate potential memory leaks.
Here is an example of how to manually dump Node.js heap memory into a file and load it on Chrome Developer Tools for inspection;
const { writeHeapSnapshot } = require('node:v8');
// Generate a heapdump for the main thread.
const mainHeapdumpFilename = writeHeapSnapshot();
console.log(`Main thread heapdump: ${mainHeapdumpFilename}`);
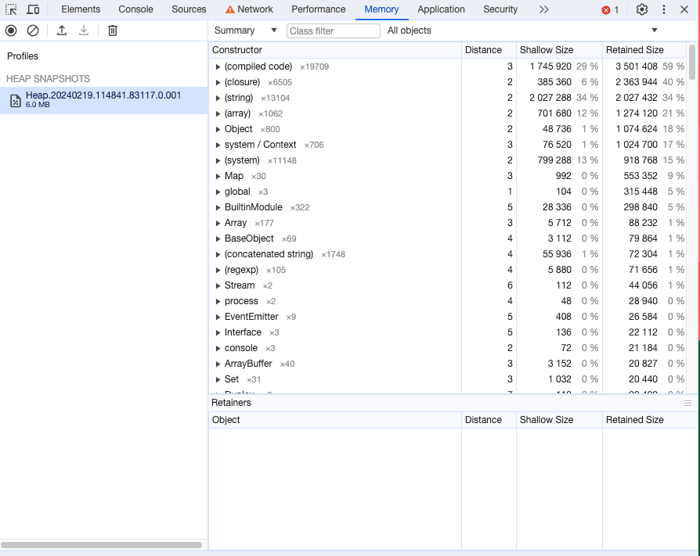
The feature of generating a heap dump on Node.js was added at v12. If you use an older version, you can install an external package heapdump
and achieve the same thing.
2. Leverage `--inspect` and Chrome Developer Tools
Activate the `--inspect` flag when running your Node.js application to enable debugging with Chrome Developer Tools. This allows you to explore memory usage, set breakpoints, and step through code to identify memory leak sources.
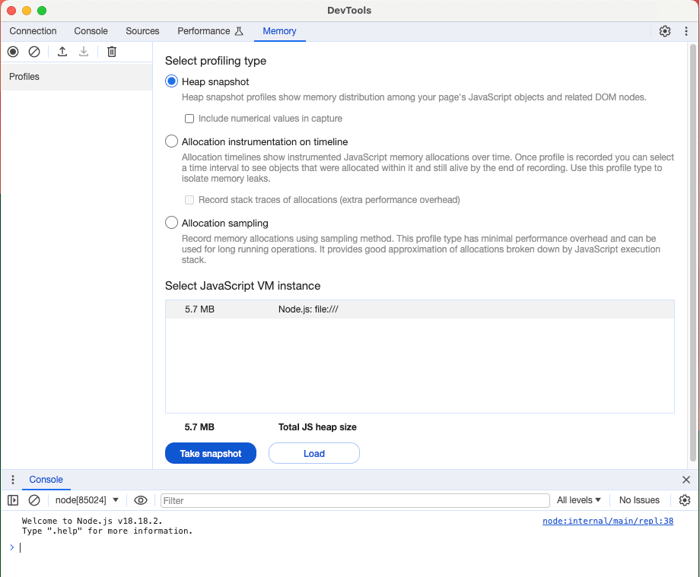
3. Memory Profilers
You can add tools like heapdump
and memwatch
to your project to capture memory usage data and analyze it over time. These tools are particularly useful for identifying patterns and trends.
const heapdump = require('heapdump');
const memwatch = require('memwatch-next');
// Create a heapdump on demand
const createHeapdump = () => {
const filename = `heapdump-${Date.now()}.heapsnapshot`;
heapdump.writeSnapshot(filename, (err, filename) => {
if (err) {
console.error('Error creating heapdump:', err);
} else {
console.log(`Heapdump written to ${filename}`);
}
});
};
// Start memory leak detection
memwatch.on('leak', (info) => {
console.error('Memory leak detected:', info);
createHeapdump(); // Create a heapdump on memory leak detection
});
4. Debugging Flags
Leverage debugging flags such as --trace-gc
when running your Node.js application to log garbage collection events and gain additional insights into memory usage.
Example log from a node.js process with --trace-gc
enabled;
> o[88065:0x138040000] 32755 ms: Scavenge 6.3 (7.1) -> 5.4 (7.1) MB, 0.4 / 0.0 ms (average mu = 0.989, current mu = 0.989) allocation failure;
Token value |
Interpretation |
88065 |
PID of the running process |
0x138040000 |
Isolate (JS heap instance) |
32755 |
The time since the process started in ms |
Scavenge |
Type / Phase of GC |
6.3 |
Heap used before GC in MB |
(7.1) |
Total heap before GC in MB |
5.4 |
Heap used after GC in MB |
(7.1) |
Total heap after GC in MB |
0.4 / 0.0 ms (average mu = 0.989, current mu = 0.989) |
Time spent in GC in ms |
allocation failure |
Reason for GC |
5. Third-party Services
Consider using third-party services like New Relic or Datadog for comprehensive monitoring and analysis of your application's memory usage.
By employing a combination of these tools and techniques, you can gain valuable insights into your application's memory behavior and pinpoint areas where memory leaks may occur.
Detecting memory leaks is only half the battle. Once you've identified potential issues, effective debugging techniques are crucial for understanding the root causes and implementing solutions.
Node.js Memory Leaks Tips
In the dynamic landscape of Node.js development, understanding and addressing memory leaks are integral to building robust and high-performance applications. We've explored the intricacies of detecting, debugging, and preventing memory leaks. As you navigate the realm of Node.js memory management, here are key takeaways:
Detection Tools Are Crucial
Heap snapshots, monitoring tools, and debugging flags are powerful allies in detecting memory leaks. Regularly employ these tools to gain insights into your application's memory usage and identify potential issues early on.
Debugging Is a Continuous Process
Debugging memory leaks is an ongoing process. Utilize debugging flags like --expose-gc
and --trace-gc
, analyze heap snapshots, and leverage monitoring tools to understand your application's memory behavior throughout its lifecycle.
Proactive Practices Mitigate Risks
Best practices such as properly closing event listeners, breaking circular references, and regular resource cleanup contribute to proactive memory management. Incorporate these practices into your development workflow to minimize the likelihood of memory leaks.
Continuous Monitoring Is Key
Implement robust monitoring strategies to continuously track memory usage patterns and identify potential anomalies. You can integrate automated tests for memory leaks into your testing pipeline for added assurance.
Real-world Examples Offer Valuable Insights
Real-world examples from companies like GitHub, PayPal, Walmart, LinkedIn, and Netflix underscore the importance of diverse strategies and tools in addressing memory leaks. Learn from these examples to enhance your own debugging and prevention practices.
Summary
Mastering Node.js memory management is an ongoing journey that requires a combination of tools, techniques, and proactive coding practices. By staying vigilant, leveraging debugging tools, and learning from real-world scenarios, you can build Node.js applications that deliver optimal performance and reliability.