Java Toolkit: Code Coverage Tools And Application Frameworks
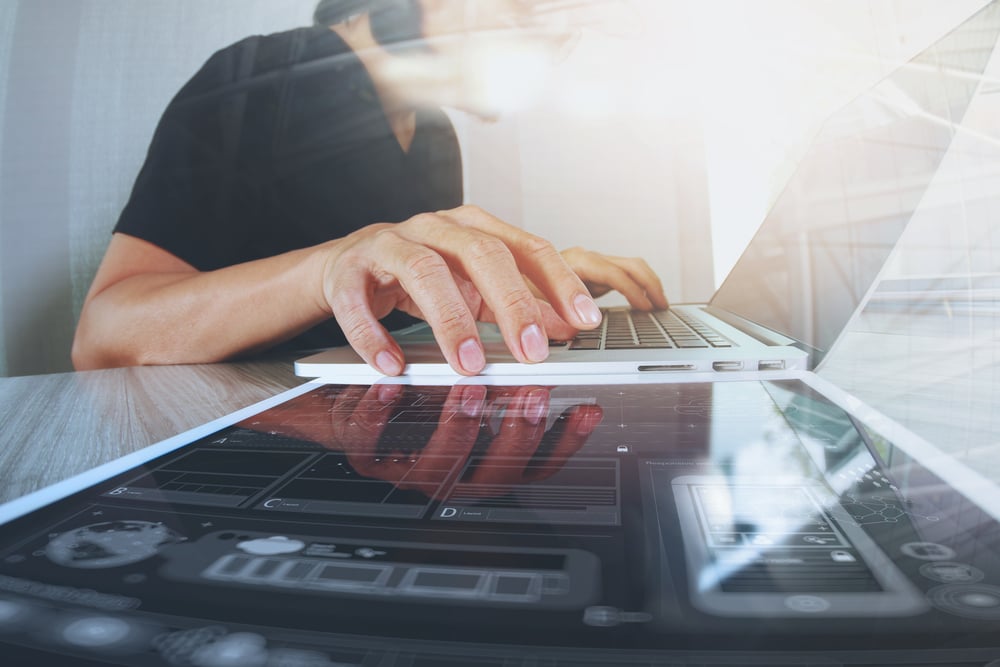
Java is a programming language that comes with lots of tools to automate, integrate, deploy, and generally ease the work of everyone involved in the process, such as Devs, QAs, and DevOps.
Trying to choose the all-time best code coverage tools for Java is difficult, because the project setup varies from one to another, but there are solutions available on the market that have advantages over the competition or are designed for a specific use case. Based on that, a recommendation can be prepared and presented to the client in the project setup phase.
Looking at the setup for backend, frontend, and mobile development, there are quite a few tools for measuring code coverage for Java, spanning to IDE (integrated development environment), version control, source code automation and management tools, databases, useful dependencies, DI (dependency injection), and inspections.
In this article, I’ll talk about the application frameworks and popular code coverage tools for Java and use cases that help develop a variety of applications.
Java version
When discussing the tools for Java-based application development, it’s important to start with the language distribution itself. The latest Java 17 LTS (long-term support) has lots of useful features, but it comes in different distributions – the one you use is down to the project setup, but the best-known ones are:
- OpenJDK 17
- Amazon Corretto 17
- RedHat build of OpenJDK 17
- Oracle Java SE 17
The important thing to consider is that some are licensed, like Oracle. The other thing to think about is where you want to deploy your service – for example with AWS (Amazon Web Services) it’s useful to use the Amazon distribution.
These factors depend on project requirements and there’s no wrong approach. Generally, I recommend starting with the default OpenJDK and adjusting if necessary.
Integrated Development Environments
There are a few IDEs to choose from. The top three IDE tools we recommend are:
- IntelliJ IDEA
- Eclipse
- NetBeans
All of these have been on the market for years now and are well-known to developers. The paid Ultimate version of IntelliJ IDEA has all you need when developing software, while the open-source Eclipse provides enormous amounts of plugins to make development easier.
When a project is just starting, it's up to the team to decide which one to use; when a project is already ongoing, I recommend sticking to the IDE the team is using. When developers use the same IDE as the rest of the team, that allows them to quickly make adjustments or fix common issues that may appear, helping the wider team work faster.
Code syntax and formatting
In addition to IDEs, it’s worth installing additional plugins that allow each developer on the team to follow the same formatting standards.
Every day, lots of changes are made to the source code repository and they can potentially create merge conflicts between developers who may have different code syntax and formatting setups in their IDE. That happens if people join the team from different projects.
Code syntax
The Checkstyle plugin allows developers to follow good practices during development, because source code is highlighted where it can be improved. All three IDEs mentioned above have their own respective Checkstyle plugin.
Formatting
Code formatting using Google Style allows you to follow the same formatting as the rest of the team. You can define your own, but Google Style is the most common.
Version control
The three most popular tools for version control are:
- Git
- Apache Subversion (svn)
- Mercurial
Git is one of the best version control tools, with its tremendous community support, distributed model, and open-source code. Largely influenced by the successful GitHub page that provides an intuitive GUI (graphical user interface), Git is generally a better choice to use over its competitors.
Git and Mercurial are quite similar in terms of the way they work. Both have a distributed model, so they don’t require a centralized server, whereas Apache Subversion (svn) follows a centralized model.
Git tracks changes at repository level and Apache Subversion (svn) at file level. Apache Subversion (svn) branching requires a lot of disk space, but is great for handling big binary files. Git offers better performance when working with smaller-sized files.
Build automation tools
To handle dependencies management, build automation, packaging, unit tests, and deployment, two major competitors come to the stage:- Gradle
- Maven
Gradle is highly customizable, but at the cost of being a more complex solution than Maven. In my opinion, there’s no clear winner in this category. That’s because projects usually follow the same setup process, and it’s more team composition and skills that matter in this instance.
Databases
Connections with databases is a key area where you should analyze the right code coverage tool to use. Specific databases – relational or not – don’t immediately indicate which tool or language is preferable, because the major players support multiple ones.
For relational databases, it could be PostgreSQL, MySQL, or Oracle DB, and for non-relational, MongoDB.
Persistence API
There’s one key player that covers the persistence layer: Hibernate.
Hibernate facilitates the connection between Java applications and the database, by providing convenient interfaces for relational databases. It also has a Hibernate OGM that does similar things, but for NoSQL databases.
Additionally, Hibernate offers the possibility to use JPQL (Java Persistence Query Language), datastore-specific native queries, and full-text queries, using Hibernate Search as an indexing engine.
Version control
After creating the database, it’s inevitably updated when new features are added. The database could be migrated or revert the changes. It’s worth considering the following two solutions when discussing evolutionary database design.
Liquibase
Liquibase is an easy-to-use tool for versioning the database model. It stores information about changes incrementally and quick model rebuilds, reverts, or updates. It’s not the best tool for data versioning or transferring, because this area adds an additional layer of complexity.
Liquibase also offers the possibility to specify changes in different formats like XML (extensible markup language) and SQL (structured query language). Changes are managed by the master changelog.
Flyway
Flyway is a tool that favors simplicity and convention over configuration. It follows linear versioning and increments on each version that’s changed. It only uses SQL to define changes and doesn’t support the creation of a snapshot of the current database or conditional deployment, whereas Liquibase does.
Useful dependencies
Developing applications creates lots of possibilities to reuse portions of code, because multiple low-level functionalities don’t change between projects. The ones mentioned below are the most useful.
Log4j2
Log4j2 is the updated version of Log4j and is a logging facade for Java applications for logging, debugging, and auditing purposes. It has great configuration possibilities, allowing you to format messages any way you like, is lightweight, and can save hours of development by providing an easy-to-use interface.
Lombok
Project Lombok offers the possibility to use multiple annotations to simplify code, by removing the necessity to write boilerplate code each time. It automatically plugs into the editor and builds tools, saving development time.
Jackson and Gson
Jackson and Gson are useful JSON (JavaScript Object Notation) to POJO (Plain Old Java Object) and the opposite representation converters. Both can also work with objects that don’t have pre-existing source code. Gson focuses on JSON, while Jackson provides conversion for multiple additional formats.
Apache Commons
Apache Commons is an open-source project containing multiple dependencies that provide reusable sources of Java code spanning bytecode, cryptographic, IO (input/output), logging, mathematical, and even CLI (command-line interface) parser libraries. Portions of code in the applications can definitely benefit from it.
DI/IoC(inversion of control) frameworks
Lots of dependency injection frameworks that support Java are available on the market; each has its own advantages and disadvantages. There are several well-established solutions as well as newcomers that look promising.
Spring
Spring is a general-purpose DI and IoC framework, with an enormous community support, multiple out-of-the-box integrations, and a mature interface. For those reasons, it’s the default choice for lots of projects.
Whenever DI or IoC topics for Java appear, Spring is mentioned. One of the disadvantages of Spring is its high memory footprint. Competitors use that to promote themselves, because it’s hard to compete with Spring’s rich integration possibilities.
Micronaut
Micronaut also has multiple integration possibilities, but it uses less memory and boots up faster, which in some cases is an advantage. It doesn’t use Java Reflection APIs (application programming interfaces) and integrates directly with the compiler using annotation processors, to generate an additional set of classes. Micronaut is well suited for microservices.
Dagger
Dagger is a fully static, compile-time DI framework for Java. Similar to Micronaut, it creates classes during the first run that work as an orchestration mechanism, wiring up initializations of components in the classes. It has the same advantages as Micronaut, without multiple integration components.
Google Guice
Google Guice is an easy-to-use DI framework. It follows a code-first approach that encourages defining injections only in Java code. It’s inspired by Spring and has similar functionality to Spring Boot Core, while being lightweight.
Inspections
Code inspection assesses the precision of code within an application or a product. It helps you develop top-quality software that wows the client and delights consumers.
Code coverage analysis
Developing high-quality applications requires following good programming principles and code style. Tools that automate those processes and advise developers regarding how their code could be improved are indispensable. We recommend the following two.
Code Climate
Code Climate is a cloud-based, automated code review tool to test coverage, maintainability, and code quality. It’s easily integrated with CI/CD orchestration tools.
SonarQube
SonarQube is similar to Code Climate. The difference mainly lies in the reporting and the way it’s presented in the UI. Aside from that, SonarQube has IDE integration and also offers on-premises installation.
Security analysis
CVE (common vulnerabilities and exposures) is a way to indicate what risks are affecting code through the dependencies referenced in the application or main code. To maintain the highest security, it’s important to look at one of the following tools.
Snyk
Snyk is a threat policy management system that integrates cloud infrastructure security into developer workflows to prevent unintended security threats in the application. It can be integrated with CI/CD pipelines and provides a way to evaluate compliance with regulatory and internal security policies.
Snyk is easy to use and has a modern UI. Through CVSS (common vulnerability scoring system), it indicates the characteristics and severity of software vulnerabilities. Snyk supports hybrid installation, on-premises, and cloud.
Sonatype Nexus IQ / Nexus Firewall
Nexus Firewall or its internal Nexus IQ tool allows you to review and understand security threats that appear in an application. Same as the above, it uses CVSS to measure threat severity and has a straightforward UI. They support installation on-premises and in the cloud, managed by Sonatype.
Code coverage tools and application frameworks for Java
Looking at the development phases and the whole ecosystem, there are lots of Java tools for code coverage measurement and application frameworks that can be incorporated into a project. Some are useful for specific use cases, while others are limited to the decision the team is making during the development phase.
When starting a project from scratch, it’s important to gather as much information as possible during the initial preparation phase, including business value, project roadmap, business deliverables, HLD (high-level design), system architecture, API principles, core data models, risks, stakeholders map, and more. Only then, is it possible to select the best code coverage tools for the project.
This article summarizes some of the best and most popular application frameworks and code coverage tools for Java. Each project and product is different, meaning tool combinations vary, depending on the scenario. We’re here to help you choose the best option.