11 Software Development Best Practices in 2024
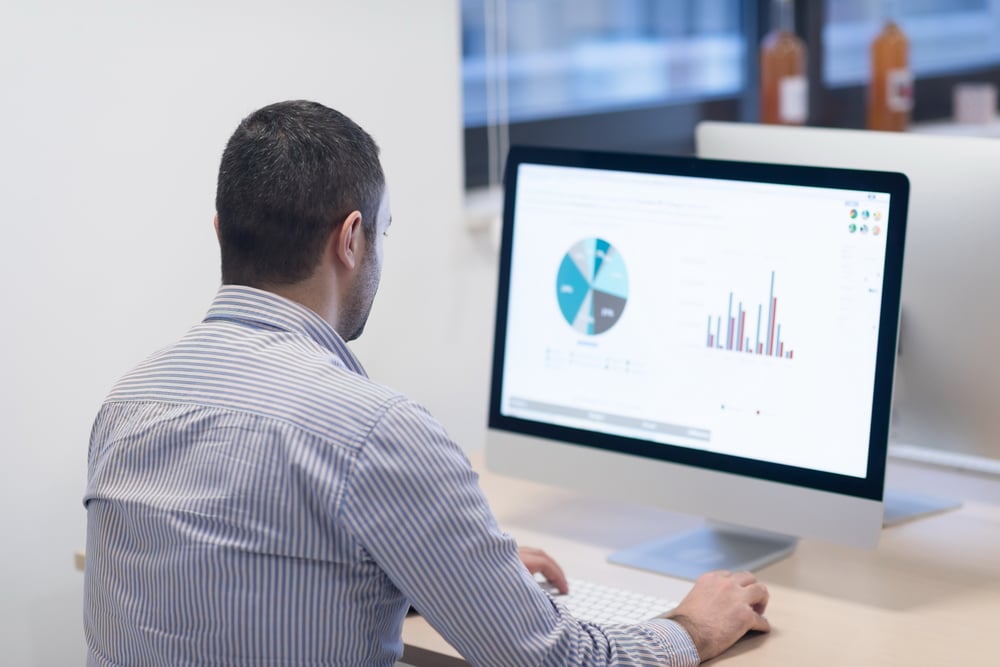
When we design and build a software product, there's a handful of software development standards and best practices to be aware of, from the YAGNI principle to paying attention to style guides.
Short summary:
- Following software development best practices leads to improved code quality, efficiency, and easier maintenance.
- Principles such as DRY and YAGNI provide guidance for effective coding and feature development.
- Robust software is achieved through thorough testing, version control, and diligent code reviews.
Following best practices is a way to improve the performance and productivity of an organization, and something we take seriously here at Netguru. When it comes to software development best practices, what should software developers consider?
From the DRY – don't repeat yourself – principle for software bugs and implementing version controls to enforcing naming conventions and designing before coding, there's no shortage of guidelines to follow. By adhering to software engineering best practices, we ensure the software and applications we create for our clients and their end users are well-designed and coded, as well as robust and thoroughly tested.
Identifying and sharing best software development practices nurtures a learning culture and fills knowledge gaps. Keeping best practices in mind also enhances efficiency, enables better decision-making, and provides employees with an internal knowledge base. Finally, costs fall and we save time, because all team members are on the same page across the software development lifecycle.
Below, we’ve created a handy listicle, outlining 10 top software development best practices we stand by, plus a range of examples.
1. DRY principle
This stands for “Don’t repeat yourself” and was formulated in 1999 by Andy Hunt and Dave Thomas. According to this principle: “Every piece of knowledge must have a single, unambiguous, authoritative representation within a system.” Fast forward to 2023, and it can still be applied to any piece of code you design.
You should always avoid knowledge duplication, meaning business logic that has a certain functionality should only appear once in your application. However, code duplication is totally acceptable – you don’t have to worry about making every single line of code unique.
For example, let’s say you have a calculation for the net price of service (applies to your suppliers) and one for the product (that customers can buy). Even though these price calculating functions may look the same, there’s no knowledge duplication, because they refer to different business applications.
If duplication of knowledge does take place, that can lead to mistakes later down the line. Why? Having to change your code in multiple places is more time-consuming and risky than working on one cohesive unit, because you may forget about all the places you need to apply changes.
2. YAGNI principle
This stands for “You Ain't Gonna Need It”: Don't write code you don't need right now, but may come in handy in the future. That future may never come, meaning you'll end up wasting time for nothing. Or, requirements for developing software may change and you'll end up needing to refactor your code. Not only will you waste your time, but other software engineers' too, since they'll be trying to work with unusable code.
If your development team’s working on a big feature, organize a planning session and split it up into smaller tasks, so that each piece of code is independent. The last thing you want is to release half-finished code that needs to be completed later on.
3. Testing best practices
Writing unit tests is one the easiest things you can do to improve code quality. Creating a working code isn’t enough: It should be tested thoroughly to ensure it always gives the desired output. Unit tests tackle small, modular pieces of code and are designed to test one functionality at a time.
When carrying out unit tests, consider different scenarios and don’t just focus on aspects where everything already works as it’s supposed to. Make your test cases fail, see what happens, and then decide if that’s what you expect/want.
For example, if your software architecture expects a user to give a numerical input, what happens if they enter a letter instead? Does the application break, throw an error, or accept the faulty input?
With that in mind, write a unit test for a fail and a success scenario. You should also aim for 100% coverage of your tests, meaning all your code should be tested.
There are libraries designed to calculate test coverage for you:
- Istanbul for Node.js
- Coverage for Python
- Serenity or JCov for Java
4. Version control
This is the practice of tracking and managing changes to software code – crucial if multiple developers are working on the same software project. New features or bug fixes can be worked on at the same time, and there’s no risk of developers overriding someone else's code. Version control tools also allow you to revert to previous versions if mistakes are made during important software development, without disrupting the work of team members.
The most popular version control systems are Git and GitHub, the web-based hosting service for Git. GitHub provides pull requests to improve version control even further – you can easily squash redundant and unclear commits, so your Git is kept clean and easily readable. Additional version control tools include CVS, SVN, and Mercurial.
5. Embrace AI Assistance
Leveraging AI tools can significantly streamline software development processes. GitHub Copilot, a prime example, is an AI-driven code completion tool developed by GitHub and OpenAI. It assists developers by providing real-time code suggestions, thereby enhancing productivity.
At Netguru, nearly 100 engineers utilize GitHub Copilot daily, cutting down development time dramatically. For instance, Copilot can generate entire lines or blocks of code, including functions and algorithms, saving time and reducing manual effort.
Moreover, it supports multiple programming languages and integrates seamlessly with popular IDEs like Visual Studio Code.
This tool not only helps in writing efficient code but also aids in learning new languages and discovering alternative coding techniques. Embracing such AI assistance enables developers to focus more on problem-solving and design, ultimately improving code quality and efficiency.
Alok Ranjan, Software Engineering Manager at Dropbox
6. Pay attention to style guides
Every computer programming language has style guides, created to make code more readable and pleasurable to work with. Using a style guide doesn’t usually affect the way the code works, so if you ignore it, your program may be fine. However, working with code that doesn’t follow best practices is generally tedious and slow.
It’s a good idea is to use a linter tool that works as a static code analyzer and uses style guides to improve code quality. Linter tools show you where your code style can be improved, and some fix minor issues for you, by changing your code while it runs.
There are multiple linter tools available for each language; the main differences are around additional features, flexibility, and the way they present data.
Examples of linter tools include:
- ESLint for JavaScript
- RuboCop for Ruby
- plus Flake8
- Pylint for Python
7. Naming conventions
There are best practices for naming variables, types, functions, and other entities in your source code and documentation that should never be ignored. It may be tempting to use letters, abbreviations, funny names, or skipping underscores, but that costs you more time and effort in writing code in the long run.
Correct naming provides meaningful information about your variable or function, allowing you to refactor easily and enhance code readability. If someone else looks at your code, they should know the purpose of a function just by reading its name – there shouldn’t be any guesswork involved. If your software project is big, it may take a long time to find a misnamed piece of existing code – an unnecessary waste of manpower.
Here’s an example:
def c(a, b):
return a * b
def count_weekly_pay(hours_worked, hourly_pay_rate):
return hours_worked * hourly_pay_rate
Both functions do exactly the same thing, but when you look at the code that uses naming conventions (2.), you instantly know its purpose and it’s easy to find by the name.
8. Design before coding
Planning is just as important as coding, and it has a huge impact on code quality. Features should be designed and researched before anyone even thinks about building them, and it should be a team effort.
There are endless software design characteristics, design patterns and tools you can implement, so discussing different options will give your team the best shot at creating an effective plan. Coding before you design usually results in tons of wasted time. Indeed, you may develop software that ends up not being the best solution, meaning work needs to be redone.
9. Don’t push too many features
It’s tempting to regularly roll out new features users will love, but that may actually make your app worse! Instead, concentrate on balancing new features with refactoring. Refactoring code is a crucial part of the software development process.
As your software grows bigger and bigger, models may change, libraries may become outdated, and code structure may evolve. If you ignore these potential issues, they end up as technical debt that grows bigger with each non-refactored version.
Actions like reducing duplicated code, simplifying complex functions, and extracting common variables and functions don't add direct business value, but they do allow software development processes to go smoothly.
10. Maintain staging and production environments
A staging environment is supposed to be as close to your production code as possible, so you can test features before making them available to users. This is an additional step in the software development lifecycle, and it makes releasing new features a longer process. With that in mind, why is it a good idea?
Staging is a place where you catch bugs or issues, before they get to the production environment and do true damage to your app. Unit tests alone aren’t enough to catch potential problems, because you can’t test integrations.
If you don’t work with a staging environment, you may release a new version of your application that doesn’t work properly. That results in a negative user experience, the need to roll back your release, potential data loss, and time and money wasted on immediate hot fixes.
11. Carry out code reviews
Code review is a process where a software developer’s code (or a pull request) is examined by another software developer. It improves the quality of the overall code, helps identify bugs, and reduces the amount of work required to optimize code later.
Analyzing a pull request or a piece of code takes time (the amount depends on the size of the feature), but that’s the only downside. It’s definitely worth it though, because this task can’t be performed by a tool or program.
Developers should check:
- Design
- Naming conventions
- Styling
- Test coverage and application
- Fulfilled requirements
- Optimization
Following software development best practices
When followed, software development best practices help produce good outcomes for both the software quality project in question and the business as a whole. They represent an optimal way to work, ensuring clients get the most out of the software we build for them.
Here at Netguru, we’re all about adhering to agreed-upon standards and guidelines, whether it’s maintaining staging and production environments, carrying out code reviews, or running unit tests. If you like the sound of the way we work, take a look at our software development services offering.