Serializable vs Parcelable
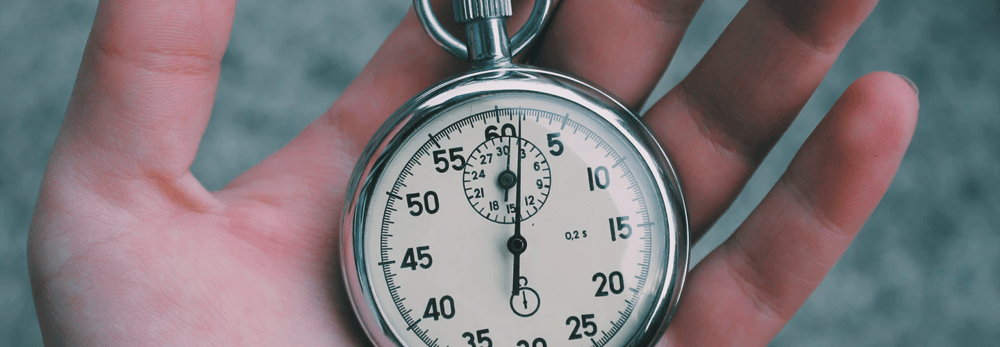
In almost every single one of them we can clearly see that Parcelable crushes Serializable in terms of speed. But implementing Parcelable always seemed like a lot of additional work. And here comes Kotlin with its magical annotations.
Introduction to @ParcelizeRemember how, whenever you wanted your object to extend from Parcelable, you had to override writeToParcel, create a new constructor, and add a static method named “CREATOR”?
data class CharacterParcelable(
val id: Int,
val image: String,
val name: String,
val type: String,
val url: String
) : Parcelable {
constructor(parcel: Parcel) : this(
parcel.readInt(),
parcel.readString()!!,
parcel.readString()!!,
parcel.readString()!!,
parcel.readString()!!
)
override fun writeToParcel(parcel: Parcel, flags: Int) {
parcel.writeInt(id)
parcel.writeString(image)
parcel.writeString(name)
parcel.writeString(type)
parcel.writeString(url)
}
override fun describeContents(): Int {
return 0
}
companion object {
@JvmField
val CREATOR = object : Parcelable.Creator<CharacterParcelable> {
override fun createFromParcel(parcel: Parcel): CharacterParcelable {
return CharacterParcelable(parcel)
}
override fun newArray(size: Int): Array<CharacterParcelable?> {
return arrayOfNulls(size)
}
}
}
}
Now all you have to do is to add @Parcelize to your class:
@Parcelize
data class CharacterParcelable(
val id: Int,
val image: String,
val name: String,
val type: String,
val url: String
) : Parcelable
Speed Test
I wanted testing to be rather simple to remove as many variables that can affect the result as possible.
val bundle = bundleOf()
for (i in 0..TESTS_COUNT) {
bundle.putParcelable("parcelable$i", parcelable)
}
for (i in 0..TESTS_COUNT) {
bundle.getParcelable<CharacterParcelable>("parcelable$i")
}
val bundle = bundleOf()
for (i in 0..TESTS_COUNT) {
bundle.putSerializable("serializable$i", serializable)
}
for (i in 0..TESTS_COUNT) {
bundle.getSerializable("serializable$i") as CharacterSerializable
}
I ran this test on four different devices with three different TEST_COUNT numbers. Our main contestants today are a Samsung S5 (4.4.2), an LG G3 (5.0.2), a Motorola Moto E2 (6.0) and a Samsung S10 (9.0). The number of iterations for each test were 1_000, 10_000 and 50_000. I repeated each test 10 times to get the best average time result.
With most of the information provided, let us just jump to what everyone is waiting for: data in charts.
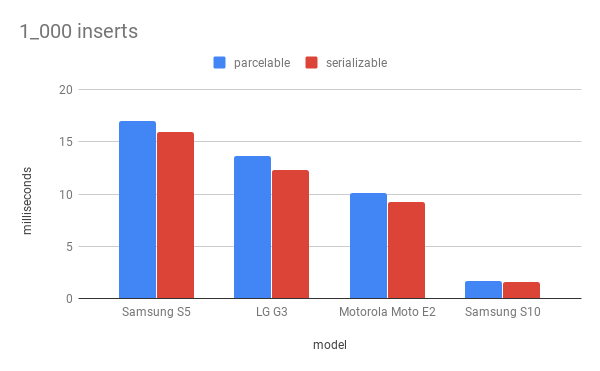
In the first test, we can see that Parcelable is slower. It’s not not by much, but there is still a difference and the result is surprising.
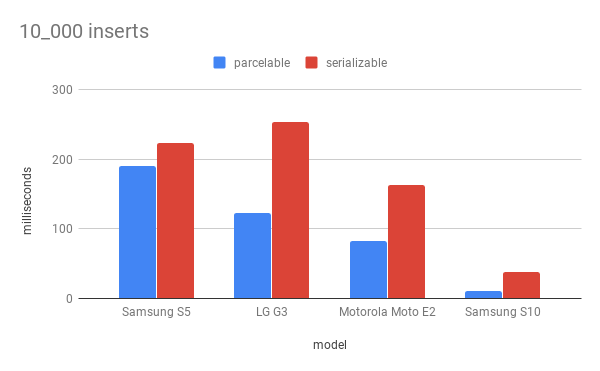
On the other hand, when we increased the number of inserts to bundle, Parcelable became visibly quicker once again - after seeing the previous chart the result is surprising.
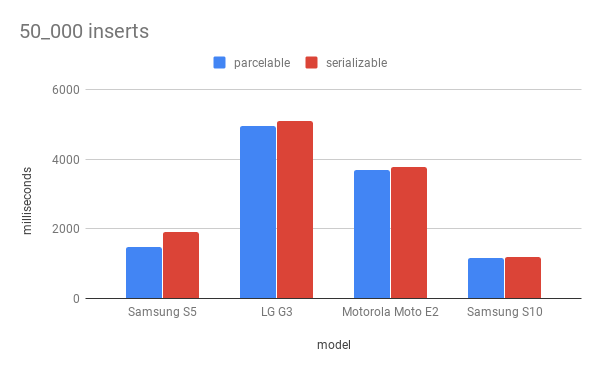
In the 50_000 inserts chart it’s very surprising that the performance of Samsung S5 has the biggest difference between times and its runtime did not increase as much as that of the LG and the Motorola.
Something Extra
While I was running my test, I thought to myself: what if Kotlin’s auto-generated code creates unnecessary cruft that slows down the process? I added
compileOptions {
sourceCompatibility JavaVersion.VERSION_1_8
targetCompatibility JavaVersion.VERSION_1_8
}
to my Gradle build file. Maybe it increases the speed of Serializable, so I implemented my own Parcelable and removed JavaVersion 1.8 compatibility and rerun the largest test on the Samsung S10.
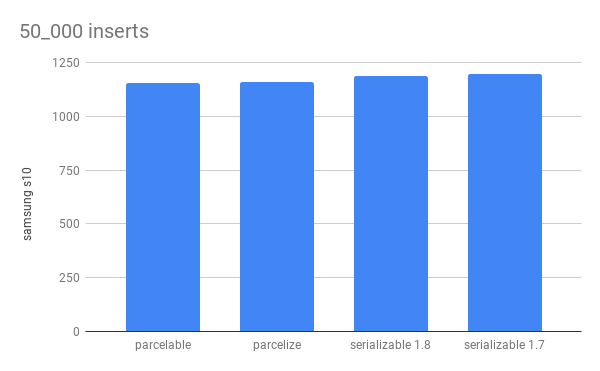
As you can see, my theory is now confirmed. But to be sure I reran this test once again on the LG G3 and all my hope was gone.
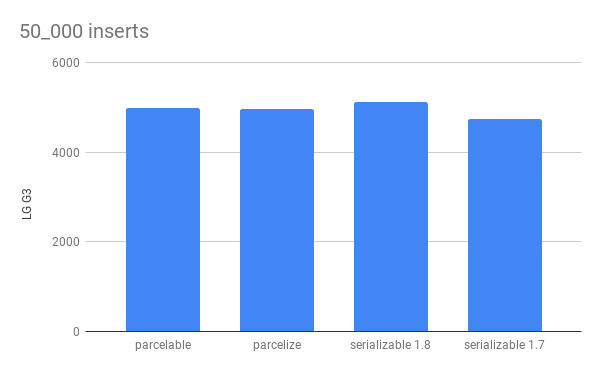
In Conclusion
As you can see, the question of comparing speed between Serializable and Parcelable cannot be answered with just “Parcelable is faster”. With the growth of the number of iterations, it is clear that Parcelize becomes more efficient. In addition, the differences in speed are more noticeable on older devices. Just remember to always change your approach according to your project’s requirements. Still, personally, I would rather use Parcelize to take advantage of reduced compile time errors from not extending Serialisation interfaces in nested objects. Oh, and I don’t even know what to think about these extra two tests.
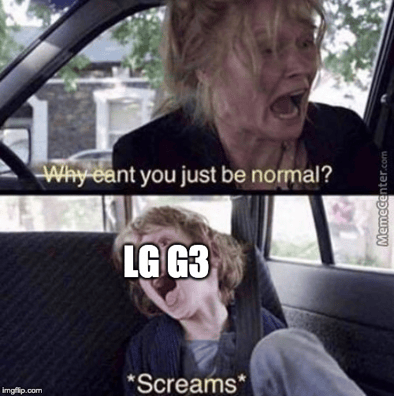